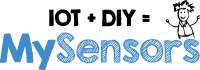 |
MySensors Library & Examples
2.3.2-62-ge298769
|
4 #include "AES_config.h"
65 byte set_key (
byte key[],
int keylen) ;
94 byte encrypt (
byte plain [N_BLOCK],
byte cipher [N_BLOCK]) ;
105 byte cbc_encrypt (
byte * plain,
byte * cipher,
int n_block,
byte iv [N_BLOCK]) ;
115 byte cbc_encrypt (
byte * plain,
byte * cipher,
int n_block) ;
130 byte decrypt (
byte cipher [N_BLOCK],
byte plain [N_BLOCK]) ;
141 byte cbc_decrypt (
byte * cipher,
byte * plain,
int n_block,
byte iv [N_BLOCK]) ;
151 byte cbc_decrypt (
byte * cipher,
byte * plain,
int n_block) ;
160 void set_IV(
unsigned long long int IVCl);
231 void printArray(
byte output[],
bool p_pad =
true);
252 void do_aes_encrypt(
byte *plain,
int size_p,
byte *cipher,
byte *key,
int bits,
byte ivl [N_BLOCK]);
263 void do_aes_encrypt(
byte *plain,
int size_p,
byte *cipher,
byte *key,
int bits);
275 void do_aes_decrypt(
byte *cipher,
int size_c,
byte *plain,
byte *key,
int bits,
byte ivl [N_BLOCK]);
286 void do_aes_decrypt(
byte *cipher,
int size_c,
byte *plain,
byte *key,
int bits);
288 #if defined(AES_LINUX)
298 byte key_sched [KEY_SCHEDULE_BYTES]
300 unsigned long long int IVC;
304 #if defined(AES_LINUX)
byte decrypt(byte cipher[N_BLOCK], byte plain[N_BLOCK])
byte cbc_decrypt(byte *cipher, byte *plain, int n_block, byte iv[N_BLOCK])
void copy_n_bytes(byte *AESt, byte *src, byte n)
void set_IV(unsigned long long int IVCl)
void do_aes_encrypt(byte *plain, int size_p, byte *cipher, byte *key, int bits, byte ivl[N_BLOCK])
byte set_key(byte key[], int keylen)
bool CheckPad(byte *in, int size)
byte cbc_encrypt(byte *plain, byte *cipher, int n_block, byte iv[N_BLOCK])
void do_aes_decrypt(byte *cipher, int size_c, byte *plain, byte *key, int bits, byte ivl[N_BLOCK])
void padPlaintext(void *in, byte *out)
void calc_size_n_pad(int p_size)
byte encrypt(byte plain[N_BLOCK], byte cipher[N_BLOCK])
void printArray(byte output[], bool p_pad=true)