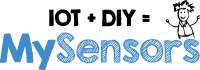 |
MySensors Library & Examples
2.3.2-62-ge298769
|
40 #define COMPARE_PH 1 // Send PH only if changed? 1 = Yes 0 = No
42 uint32_t SLEEP_TIME = 60000;
44 bool receivedConfig =
false;
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void presentation()
Node presentation.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
bool send(MyMessage &msg, const bool requestEcho=false)
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
int8_t sleep(const uint32_t sleepingMS, const bool smartSleep=false)
API declaration for MySensors.
MyMessage is used to create, manipulate, send and read MySensors messages.