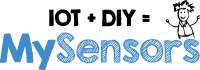 |
MySensors Library & Examples
2.3.2-62-ge298769
|
33 #define MY_PASSIVE_NODE
36 #define MY_NODE_ID 100
47 #define CHILD_ID 0 // Id of the sensor child
68 send(msg.
set(25.0+random(0,30)/10.0,2));
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void presentation()
Node presentation.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
bool send(MyMessage &msg, const bool requestEcho=false)
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
int8_t sleep(const uint32_t sleepingMS, const bool smartSleep=false)
API declaration for MySensors.
MyMessage is used to create, manipulate, send and read MySensors messages.