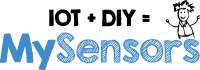 |
MySensors Library & Examples
2.3.2-62-ge298769
|
41 #define MY_REPEATER_FEATURE
45 #define RELAY_PIN 4 // Arduino Digital I/O pin number for first relay (second on pin+1 etc)
46 #define NUMBER_OF_RELAYS 1 // Total number of attached relays
47 #define RELAY_ON 1 // GPIO value to write to turn on attached relay
48 #define RELAY_OFF 0 // GPIO value to write to turn off attached relay
86 if (message.
getType()==V_STATUS) {
88 digitalWrite(message.
getSensor()-1+RELAY_PIN, message.
getBool()?RELAY_ON:RELAY_OFF);
92 Serial.print(
"Incoming change for sensor:");
94 Serial.print(
", New status: ");
95 Serial.println(message.
getBool());
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
uint8_t getSensor(void) const
Get sensor ID of message.
uint8_t sensor
8 bit - Id of sensor that this message concerns.
void receive(const MyMessage &message)
Callback for incoming messages.
uint8_t loadState(const uint8_t pos)
void presentation()
Node presentation.
void saveState(const uint8_t pos, const uint8_t value)
void before()
Called before node initialises.
uint8_t getType(void) const
Get message type.
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
API declaration for MySensors.
bool getBool(void) const
Get bool payload.
MyMessage is used to create, manipulate, send and read MySensors messages.