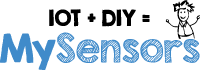 |
MySensors Library & Examples
2.3.2
|
49 #define SN "DimmableLED"
52 #define LED_PIN 3 // Arduino pin attached to MOSFET Gate pin
53 #define FADE_DELAY 10 // Delay in ms for each percentage fade up/down (10ms = 1s full-range dim)
55 static int16_t currentLevel = 0;
88 if (message.
getType() == V_LIGHT || message.
getType() == V_DIMMER) {
91 int requestedLevel = atoi( message.
data );
94 requestedLevel *= ( message.
getType() == V_LIGHT ? 100 : 1 );
97 requestedLevel = requestedLevel > 100 ? 100 : requestedLevel;
98 requestedLevel = requestedLevel < 0 ? 0 : requestedLevel;
100 Serial.print(
"Changing level to " );
101 Serial.print( requestedLevel );
102 Serial.print(
", from " );
103 Serial.println( currentLevel );
105 fadeToLevel( requestedLevel );
108 send(lightMsg.
set(currentLevel > 0));
111 send( dimmerMsg.
set(currentLevel) );
120 void fadeToLevel(
int toLevel )
123 int delta = ( toLevel - currentLevel ) < 0 ? -1 : 1;
125 while ( currentLevel != toLevel ) {
126 currentLevel += delta;
127 analogWrite( LED_PIN, (
int)(currentLevel / 100. * 255) );
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void receive(const MyMessage &message)
Callback for incoming messages.
void presentation()
Node presentation.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
bool send(MyMessage &msg, const bool requestEcho=false)
uint8_t getType(void) const
Get message type.
bool request(const uint8_t childSensorId, const uint8_t variableType, const uint8_t destination=GATEWAY_ADDRESS)
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
API declaration for MySensors.
MyMessage is used to create, manipulate, send and read MySensors messages.