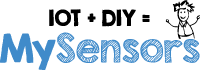 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
56 #define FLASH_ERASE_CYCLES 20000
57 #define FLASH_PAGE_SIZE 1024
58 #define FLASH_ERASE_PAGE_TIME 23
59 #define FLASH_SUPPORTS_RANDOM_WRITE true
60 #define FLASH_WRITES_PER_WORD 2
61 #define FLASH_WRITES_PER_PAGE 512
63 #define FLASH_ERASE_CYCLES 10000
64 #define FLASH_PAGE_SIZE 4096
65 #define FLASH_ERASE_PAGE_TIME 90
66 #define FLASH_SUPPORTS_RANDOM_WRITE true
67 #define FLASH_WRITES_PER_WORD 32
68 #define FLASH_WRITES_PER_PAGE 181
69 #elif defined(NRF52840)
70 #define FLASH_ERASE_CYCLES 10000
71 #define FLASH_PAGE_SIZE 4096
72 #define FLASH_ERASE_PAGE_TIME 90
73 #define FLASH_SUPPORTS_RANDOM_WRITE true
74 #define FLASH_WRITES_PER_WORD 2
75 #define FLASH_WRITES_PER_PAGE 403
77 #define FLASH_ERASE_CYCLES 10000
78 #define FLASH_PAGE_SIZE 4096
79 #define FLASH_ERASE_PAGE_TIME 100
80 //#define FLASH_SUPPORTS_RANDOM_WRITE true
81 #define FLASH_WRITES_PER_WORD 1
82 #warning "Unknown platform. Please check the code."
144 void erase(uint32_t *address,
size_t size);
154 void write(uint32_t *address, uint32_t value);
161 void write_block(uint32_t *dst_address, uint32_t *src_address,
162 uint16_t word_count);
166 void wait_for_ready();
173 #include "hal/architecture/NRF5/drivers/Flash.cpp"
175 #error "Unsupported platform."
This class provides low-level access to internal Flash memory.
FlashClass Flash
extern FlashClass
void write(uint32_t *address, uint32_t value)
uint32_t * page_address(size_t page)
uint32_t page_size() const
uint32_t specified_erase_cycles() const
uint8_t page_size_bits() const
void write_block(uint32_t *dst_address, uint32_t *src_address, uint16_t word_count)
uint32_t page_count() const
uint32_t * top_app_page_address()
void erase(uint32_t *address, size_t size)