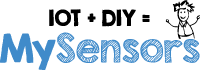 |
MySensors Library & Examples
2.3.2
|
49 uint8_t* raw_address()
51 return _address.bytes;
67 IPAddress(uint8_t first_octet, uint8_t second_octet, uint8_t third_octet, uint8_t fourth_octet);
79 explicit IPAddress(
const uint8_t *address);
100 operator uint32_t()
const
102 return _address.dword;
110 return _address.dword == addr._address.
dword;
118 return _address.dword == addr;
133 return _address.bytes[index];
141 return _address.bytes[index];
std::string toString()
Convert the IP address to a string.
bool fromString(const char *address)
Set the IP from a array of characters.
uint8_t & operator[](int index)
Overloaded index operator.
bool operator==(uint32_t addr) const
Overloaded cast operator.
IPAddress()
IPAddress constructor.
uint8_t bytes[4]
IPv4 address as an array.
bool operator==(const IPAddress &addr) const
Overloaded cast operator.
uint8_t operator[](int index) const
Overloaded index operator.
IPAddress & operator=(const uint8_t *address)
Overloaded copy operators.
bool fromString(const std::string &address)
Set the IP from a string class type.
A class to make it easier to handle and pass around IP addresses.
uint32_t dword
IPv4 address in 32 bits format.