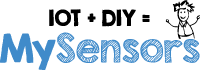 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
57 #ifndef MyOTAFirmwareUpdate_h
58 #define MyOTAFirmwareUpdate_h
61 #ifdef MCUBOOT_PRESENT
62 #include "generated_dts_board.h"
63 #define FIRMWARE_PROTOCOL_31
68 #if MAX_PAYLOAD_SIZE >= 22
69 #define FIRMWARE_BLOCK_SIZE (16u)
71 #define FIRMWARE_BLOCK_SIZE (8u)
72 #ifndef FIRMWARE_PROTOCOL_31
73 #define FIRMWARE_PROTOCOL_31
77 #define MY_OTA_RETRY (5u)
79 #ifndef MY_OTA_RETRY_DELAY
80 #define MY_OTA_RETRY_DELAY (500u)
82 #ifndef MCUBOOT_PRESENT
83 #define FIRMWARE_START_OFFSET (10u)
85 #define FIRMWARE_START_OFFSET (FLASH_AREA_IMAGE_1_OFFSET_0)
88 #define MY_OTA_BOOTLOADER_MAJOR_VERSION (3u)
89 #ifdef FIRMWARE_PROTOCOL_31
90 #define MY_OTA_BOOTLOADER_MINOR_VERSION (1u)
92 #define MY_OTA_BOOTLOADER_MINOR_VERSION (0u)
94 #define MY_OTA_BOOTLOADER_VERSION (MY_OTA_BOOTLOADER_MINOR_VERSION * 256 + MY_OTA_BOOTLOADER_MAJOR_VERSION)
96 #if defined(MY_DEBUG_VERBOSE_OTA_UPDATE)
97 #define OTA_DEBUG(x,...) DEBUG_OUTPUT(x, ##__VA_ARGS__)
98 //#define OTA_EXTRA_FLASH_DEBUG
100 #define OTA_DEBUG(x,...)
103 #if defined(DOXYGEN) && !defined(FIRMWARE_PROTOCOL_31)
111 #define FIRMWARE_PROTOCOL_31
133 #ifdef FIRMWARE_PROTOCOL_31
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
uint8_t type
8 bit - Type varies depending on command
uint16_t block
Block index.
uint32_t img_build_num
mcuboot build_num attribute, when protocol version >= 3.1 is reported
uint16_t number_of_blocks
Number of blocks to fill with data.
LOCAL bool transportIsValidFirmware(void)
Validate uploaded FW CRC.
LOCAL void firmwareOTAUpdateRequest(void)
Handle OTA FW update requests.
uint16_t img_revision
mcuboot revision attribute, when protocol version >= 3.1 is reported
LOCAL void presentBootloaderInformation(void)
Present bootloader/FW information upon startup.
uint16_t version
Version of config.
uint8_t img_commited
mcuboot image_ok attribute commited firmware=0x01(mcuboot)|0x02(DualOptiboot), when protocol version ...
uint16_t type
Type of config.
struct @4::@5 __attribute__
Doxygen will complain without this comment.
LOCAL bool firmwareOTAUpdateProcess(void)
Handle OTA FW update responses.
LOCAL void readFirmwareSettings(void)
Read firmware settings from EEPROM.
uint16_t blocks
Number of blocks.
uint16_t BLVersion
Bootloader version.
uint16_t crc
CRC of block data.
uint8_t blockSize
Blocksize, when protocol version >= 3.1 is reported. Otherwhise the blocksize is 16.
#define FIRMWARE_BLOCK_SIZE
Size of each firmware block.