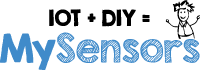 |
MySensors Library & Examples
2.3.2
|
31 #define CHILD_SENSOR_ID 0
32 #define ACTUATOR_ID 200
35 #if defined(SIGNING_ENABLED)
37 #define MY_SIGNING_SOFT
39 #define MY_DEBUG_VERBOSE_SIGNING
45 static const uint32_t trigger_ms = 10000;
48 MyMessage msgGeneral(CHILD_SENSOR_ID, V_STATUS);
49 uint32_t lastTrigger = 0;
50 bool actuatorStatus =
false;
55 present(CHILD_SENSOR_ID, S_BINARY,
"Remote");
60 #if defined(SIGNING_ENABLED)
61 SET_SIGN(ACTUATOR_ID);
67 if (millis() - lastTrigger > trigger_ms) {
68 lastTrigger = millis();
72 send(msgGeneral.
set(actuatorStatus));
74 actuatorStatus ^=
true;
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void presentation(void)
Node presentation.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
bool send(MyMessage &msg, const bool requestEcho=false)
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
MyMessage & setDestination(const uint8_t destinationId)
Set final destination node id for this message.
API declaration for MySensors.
MyMessage is used to create, manipulate, send and read MySensors messages.