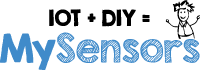 |
MySensors Library & Examples
2.3.2
|
61 LOG(F(
"\n%sReady.\n"), nodeTypeAsCharRepresentation(
getNodeId()));
71 byte inChar = Serial.
read();
75 if (inChar ==
'T' || inChar ==
't') {
76 LOG(F(
"T received - starting test...\n"));
78 msg.
sender = (node == YING ? YANG : YING);
79 sendPingOrPongResponse( msg );
80 }
else if (inChar ==
'0' or inChar ==
'1') {
81 byte nodeID = 200 + (inChar -
'0');
84 LOG(
"Invalid input\n");
92 LOG(F(
"Received %s from %s\n"), msgTypeAsCharRepresentation((mysensors_data_t)message.
getType()),
93 nodeTypeAsCharRepresentation(message.
sender));
96 sendPingOrPongResponse( message );
99 void sendPingOrPongResponse(
MyMessage msg )
104 LOG(F(
"Sending %s to %s\n"), msgTypeAsCharRepresentation( (mysensors_data_t)response.
getType() ),
105 nodeTypeAsCharRepresentation(msg.
sender));
108 response.
set( (uint32_t)millis() );
113 void setNodeId(
byte nodeID)
115 LOG(F(
"Setting node id to: %i.\n***Please restart the node for changes to take effect.\n"), nodeID);
119 const char * msgTypeAsCharRepresentation( mysensors_data_t mType )
121 return mType == V_VAR1 ?
"Ping" :
"Pong";
124 const char * nodeTypeAsCharRepresentation( uint8_t node )
126 return node == YING ?
"Ying Node" :
"Yang Node";
uint8_t sender
8 bit - Id of sender node (origin)
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void receive(const MyMessage &message)
Callback for incoming messages.
void presentation()
Node presentation.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
bool send(MyMessage &msg, const bool requestEcho=false)
uint8_t getType(void) const
Get message type.
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
void setup()
Called after node initialises but before main loop.
MyMessage & setDestination(const uint8_t destinationId)
Set final destination node id for this message.
#define EEPROM_NODE_ID_ADDRESS
Address node ID.
int available()
This function does nothing.
API declaration for MySensors.
int read()
Reads 1 key pressed from the keyboard.
MyMessage is used to create, manipulate, send and read MySensors messages.