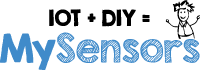 |
MySensors Library & Examples
2.3.2
|
27 #include <linux/spi/spidev.h>
29 #define SPI_HAS_TRANSACTION
32 #define LSBFIRST SPI_LSB_FIRST
34 #define SPI_CLOCK_BASE 16000000 // 16Mhz
36 #define SPI_CLOCK_DIV1 1
37 #define SPI_CLOCK_DIV2 2
38 #define SPI_CLOCK_DIV4 4
39 #define SPI_CLOCK_DIV8 8
40 #define SPI_CLOCK_DIV16 16
41 #define SPI_CLOCK_DIV32 32
42 #define SPI_CLOCK_DIV64 64
43 #define SPI_CLOCK_DIV128 128
44 #define SPI_CLOCK_DIV256 256
47 #define SPI_MODE0 SPI_MODE_0
48 #define SPI_MODE1 SPI_MODE_1
49 #define SPI_MODE2 SPI_MODE_2
50 #define SPI_MODE3 SPI_MODE_3
52 #ifndef SPI_SPIDEV_DEVICE
53 #define SPI_SPIDEV_DEVICE "/dev/spidev0.0"
57 const uint8_t SS = 24;
58 const uint8_t MOSI = 19;
59 const uint8_t MISO = 21;
60 const uint8_t SCK = 23;
74 init(SPI_CLOCK_BASE, MSBFIRST, SPI_MODE0);
85 init(
clock, bitOrder, dataMode);
100 void init(uint32_t clk, uint8_t bitOrder, uint8_t dataMode)
124 static void begin(
int busNo=0);
167 static void transfernb(
char* tbuf,
char* rbuf, uint32_t len);
174 static void transfern(
char* buf, uint32_t len);
199 static uint8_t initialized;
201 static std::string device;
203 static uint32_t speed;
204 static uint8_t bit_order;
205 static struct spi_ioc_transfer tr;
static void setBitOrder(uint8_t bit_order)
Sets the SPI bit order.
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
static void end()
End SPI operations.
static void endTransaction()
End SPI transaction.
static void transfern(char *buf, uint32_t len)
Transfer a buffer of data without an rx buffer.
static void transfernb(char *tbuf, char *rbuf, uint32_t len)
Transfer a buffer of data.
SPIDEVClass()
SPIDEVClass constructor.
SPISettings(uint32_t clock, uint8_t bitOrder, uint8_t dataMode)
SPISettings constructor.
static void usingInterrupt(uint8_t interruptNumber)
Not implemented.
uint8_t border
SPI bit order.
SPISettings()
SPISettings constructor.
static void setDataMode(uint8_t data_mode)
Sets the SPI data mode.
static void beginTransaction(SPISettings settings)
Start SPI transaction.
uint8_t dmode
SPI data mode.
static uint8_t transfer(uint8_t data)
Transfer a single byte.
static void chipSelect(int csn_chip)
Sets the chip select pin.
static void begin(int busNo=0)
Start SPI operations.
static void setClockDivider(uint16_t divider)
Sets the SPI clock divider and therefore the SPI clock speed.
static void notUsingInterrupt(uint8_t interruptNumber)
Not implemented.