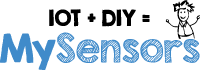 |
MySensors Library & Examples
2.3.2
|
56 int init(
const char *fileName,
size_t length);
69 void readBlock(
void* buf,
void* addr,
size_t length);
77 void writeBlock(
void* buf,
void* addr,
size_t length);
uint8_t readByte(int addr)
Read a byte from eeprom.
int init(const char *fileName, size_t length)
Initializes the eeprom class.
void destroy()
Clear all allocated memory variables.
~SoftEeprom()
SoftEeprom destructor.
void readBlock(void *buf, void *addr, size_t length)
Read a block of bytes from eeprom.
SoftEeprom & operator=(const SoftEeprom &other)
Overloaded assign operator.
void writeBlock(void *buf, void *addr, size_t length)
Write a block of bytes to eeprom.
SoftEeprom()
SoftEeprom constructor.
void writeByte(int addr, uint8_t value)
Write a byte to eeprom.