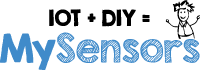 |
MySensors Library & Examples
2.3.2
|
555 #define BCM2835_VERSION 10060
563 #define BCM2835_HAVE_DMB
578 #define MIN(a, b) (a < b ? a : b)
582 #define BCM2835_CORE_CLK_HZ 250000000
585 #define BMC2835_RPI2_DT_FILENAME "/proc/device-tree/soc/ranges"
594 #define BCM2835_PERI_BASE 0x20000000
596 #define BCM2835_PERI_SIZE 0x01000000
598 #define BCM2835_RPI2_PERI_BASE 0x3F000000
600 #define BCM2835_RPI4_PERI_BASE 0xFE000000
602 #define BCM2835_RPI4_PERI_SIZE 0x01800000
607 #define BCM2835_ST_BASE 0x3000
609 #define BCM2835_GPIO_PADS 0x100000
611 #define BCM2835_CLOCK_BASE 0x101000
613 #define BCM2835_GPIO_BASE 0x200000
615 #define BCM2835_SPI0_BASE 0x204000
617 #define BCM2835_BSC0_BASE 0x205000
619 #define BCM2835_GPIO_PWM 0x20C000
621 #define BCM2835_AUX_BASE 0x215000
623 #define BCM2835_SPI1_BASE 0x215080
625 #define BCM2835_SPI2_BASE 0x2150C0
627 #define BCM2835_BSC1_BASE 0x804000
708 #define BCM2835_PAGE_SIZE (4*1024)
710 #define BCM2835_BLOCK_SIZE (4*1024)
720 #define BCM2835_GPFSEL0 0x0000
721 #define BCM2835_GPFSEL1 0x0004
722 #define BCM2835_GPFSEL2 0x0008
723 #define BCM2835_GPFSEL3 0x000c
724 #define BCM2835_GPFSEL4 0x0010
725 #define BCM2835_GPFSEL5 0x0014
726 #define BCM2835_GPSET0 0x001c
727 #define BCM2835_GPSET1 0x0020
728 #define BCM2835_GPCLR0 0x0028
729 #define BCM2835_GPCLR1 0x002c
730 #define BCM2835_GPLEV0 0x0034
731 #define BCM2835_GPLEV1 0x0038
732 #define BCM2835_GPEDS0 0x0040
733 #define BCM2835_GPEDS1 0x0044
734 #define BCM2835_GPREN0 0x004c
735 #define BCM2835_GPREN1 0x0050
736 #define BCM2835_GPFEN0 0x0058
737 #define BCM2835_GPFEN1 0x005c
738 #define BCM2835_GPHEN0 0x0064
739 #define BCM2835_GPHEN1 0x0068
740 #define BCM2835_GPLEN0 0x0070
741 #define BCM2835_GPLEN1 0x0074
742 #define BCM2835_GPAREN0 0x007c
743 #define BCM2835_GPAREN1 0x0080
744 #define BCM2835_GPAFEN0 0x0088
745 #define BCM2835_GPAFEN1 0x008c
746 #define BCM2835_GPPUD 0x0094
747 #define BCM2835_GPPUDCLK0 0x0098
748 #define BCM2835_GPPUDCLK1 0x009c
751 #define BCM2835_GPPUPPDN0 0x00e4
752 #define BCM2835_GPPUPPDN1 0x00e8
753 #define BCM2835_GPPUPPDN2 0x00ec
754 #define BCM2835_GPPUPPDN3 0x00f0
781 #define BCM2835_GPIO_PUD_ERROR 0x08
784 #define BCM2835_PADS_GPIO_0_27 0x002c
785 #define BCM2835_PADS_GPIO_28_45 0x0030
786 #define BCM2835_PADS_GPIO_46_53 0x0034
789 #define BCM2835_PAD_PASSWRD (0x5A << 24)
790 #define BCM2835_PAD_SLEW_RATE_UNLIMITED 0x10
791 #define BCM2835_PAD_HYSTERESIS_ENABLED 0x08
792 #define BCM2835_PAD_DRIVE_2mA 0x00
793 #define BCM2835_PAD_DRIVE_4mA 0x01
794 #define BCM2835_PAD_DRIVE_6mA 0x02
795 #define BCM2835_PAD_DRIVE_8mA 0x03
796 #define BCM2835_PAD_DRIVE_10mA 0x04
797 #define BCM2835_PAD_DRIVE_12mA 0x05
798 #define BCM2835_PAD_DRIVE_14mA 0x06
799 #define BCM2835_PAD_DRIVE_16mA 0x07
909 #define BCM2835_AUX_IRQ 0x0000
910 #define BCM2835_AUX_ENABLE 0x0004
912 #define BCM2835_AUX_ENABLE_UART1 0x01
913 #define BCM2835_AUX_ENABLE_SPI0 0x02
914 #define BCM2835_AUX_ENABLE_SPI1 0x04
917 #define BCM2835_AUX_SPI_CNTL0 0x0000
918 #define BCM2835_AUX_SPI_CNTL1 0x0004
919 #define BCM2835_AUX_SPI_STAT 0x0008
920 #define BCM2835_AUX_SPI_PEEK 0x000C
921 #define BCM2835_AUX_SPI_IO 0x0020
922 #define BCM2835_AUX_SPI_TXHOLD 0x0030
924 #define BCM2835_AUX_SPI_CLOCK_MIN 30500
925 #define BCM2835_AUX_SPI_CLOCK_MAX 125000000
927 #define BCM2835_AUX_SPI_CNTL0_SPEED 0xFFF00000
928 #define BCM2835_AUX_SPI_CNTL0_SPEED_MAX 0xFFF
929 #define BCM2835_AUX_SPI_CNTL0_SPEED_SHIFT 20
931 #define BCM2835_AUX_SPI_CNTL0_CS0_N 0x000C0000
932 #define BCM2835_AUX_SPI_CNTL0_CS1_N 0x000A0000
933 #define BCM2835_AUX_SPI_CNTL0_CS2_N 0x00060000
935 #define BCM2835_AUX_SPI_CNTL0_POSTINPUT 0x00010000
936 #define BCM2835_AUX_SPI_CNTL0_VAR_CS 0x00008000
937 #define BCM2835_AUX_SPI_CNTL0_VAR_WIDTH 0x00004000
938 #define BCM2835_AUX_SPI_CNTL0_DOUTHOLD 0x00003000
939 #define BCM2835_AUX_SPI_CNTL0_ENABLE 0x00000800
940 #define BCM2835_AUX_SPI_CNTL0_CPHA_IN 0x00000400
941 #define BCM2835_AUX_SPI_CNTL0_CLEARFIFO 0x00000200
942 #define BCM2835_AUX_SPI_CNTL0_CPHA_OUT 0x00000100
943 #define BCM2835_AUX_SPI_CNTL0_CPOL 0x00000080
944 #define BCM2835_AUX_SPI_CNTL0_MSBF_OUT 0x00000040
945 #define BCM2835_AUX_SPI_CNTL0_SHIFTLEN 0x0000003F
947 #define BCM2835_AUX_SPI_CNTL1_CSHIGH 0x00000700
948 #define BCM2835_AUX_SPI_CNTL1_IDLE 0x00000080
949 #define BCM2835_AUX_SPI_CNTL1_TXEMPTY 0x00000040
950 #define BCM2835_AUX_SPI_CNTL1_MSBF_IN 0x00000002
951 #define BCM2835_AUX_SPI_CNTL1_KEEP_IN 0x00000001
953 #define BCM2835_AUX_SPI_STAT_TX_LVL 0xFF000000
954 #define BCM2835_AUX_SPI_STAT_RX_LVL 0x00FF0000
955 #define BCM2835_AUX_SPI_STAT_TX_FULL 0x00000400
956 #define BCM2835_AUX_SPI_STAT_TX_EMPTY 0x00000200
957 #define BCM2835_AUX_SPI_STAT_RX_FULL 0x00000100
958 #define BCM2835_AUX_SPI_STAT_RX_EMPTY 0x00000080
959 #define BCM2835_AUX_SPI_STAT_BUSY 0x00000040
960 #define BCM2835_AUX_SPI_STAT_BITCOUNT 0x0000003F
966 #define BCM2835_SPI0_CS 0x0000
967 #define BCM2835_SPI0_FIFO 0x0004
968 #define BCM2835_SPI0_CLK 0x0008
969 #define BCM2835_SPI0_DLEN 0x000c
970 #define BCM2835_SPI0_LTOH 0x0010
971 #define BCM2835_SPI0_DC 0x0014
974 #define BCM2835_SPI0_CS_LEN_LONG 0x02000000
975 #define BCM2835_SPI0_CS_DMA_LEN 0x01000000
976 #define BCM2835_SPI0_CS_CSPOL2 0x00800000
977 #define BCM2835_SPI0_CS_CSPOL1 0x00400000
978 #define BCM2835_SPI0_CS_CSPOL0 0x00200000
979 #define BCM2835_SPI0_CS_RXF 0x00100000
980 #define BCM2835_SPI0_CS_RXR 0x00080000
981 #define BCM2835_SPI0_CS_TXD 0x00040000
982 #define BCM2835_SPI0_CS_RXD 0x00020000
983 #define BCM2835_SPI0_CS_DONE 0x00010000
984 #define BCM2835_SPI0_CS_TE_EN 0x00008000
985 #define BCM2835_SPI0_CS_LMONO 0x00004000
986 #define BCM2835_SPI0_CS_LEN 0x00002000
987 #define BCM2835_SPI0_CS_REN 0x00001000
988 #define BCM2835_SPI0_CS_ADCS 0x00000800
989 #define BCM2835_SPI0_CS_INTR 0x00000400
990 #define BCM2835_SPI0_CS_INTD 0x00000200
991 #define BCM2835_SPI0_CS_DMAEN 0x00000100
992 #define BCM2835_SPI0_CS_TA 0x00000080
993 #define BCM2835_SPI0_CS_CSPOL 0x00000040
994 #define BCM2835_SPI0_CS_CLEAR 0x00000030
995 #define BCM2835_SPI0_CS_CLEAR_RX 0x00000020
996 #define BCM2835_SPI0_CS_CLEAR_TX 0x00000010
997 #define BCM2835_SPI0_CS_CPOL 0x00000008
998 #define BCM2835_SPI0_CS_CPHA 0x00000004
999 #define BCM2835_SPI0_CS_CS 0x00000003
1067 #define BCM2835_BSC_C 0x0000
1068 #define BCM2835_BSC_S 0x0004
1069 #define BCM2835_BSC_DLEN 0x0008
1070 #define BCM2835_BSC_A 0x000c
1071 #define BCM2835_BSC_FIFO 0x0010
1072 #define BCM2835_BSC_DIV 0x0014
1073 #define BCM2835_BSC_DEL 0x0018
1074 #define BCM2835_BSC_CLKT 0x001c
1077 #define BCM2835_BSC_C_I2CEN 0x00008000
1078 #define BCM2835_BSC_C_INTR 0x00000400
1079 #define BCM2835_BSC_C_INTT 0x00000200
1080 #define BCM2835_BSC_C_INTD 0x00000100
1081 #define BCM2835_BSC_C_ST 0x00000080
1082 #define BCM2835_BSC_C_CLEAR_1 0x00000020
1083 #define BCM2835_BSC_C_CLEAR_2 0x00000010
1084 #define BCM2835_BSC_C_READ 0x00000001
1087 #define BCM2835_BSC_S_CLKT 0x00000200
1088 #define BCM2835_BSC_S_ERR 0x00000100
1089 #define BCM2835_BSC_S_RXF 0x00000080
1090 #define BCM2835_BSC_S_TXE 0x00000040
1091 #define BCM2835_BSC_S_RXD 0x00000020
1092 #define BCM2835_BSC_S_TXD 0x00000010
1093 #define BCM2835_BSC_S_RXR 0x00000008
1094 #define BCM2835_BSC_S_TXW 0x00000004
1095 #define BCM2835_BSC_S_DONE 0x00000002
1096 #define BCM2835_BSC_S_TA 0x00000001
1098 #define BCM2835_BSC_FIFO_SIZE 16
1132 #define BCM2835_ST_CS 0x0000
1133 #define BCM2835_ST_CLO 0x0004
1134 #define BCM2835_ST_CHI 0x0008
1140 #define BCM2835_PWM_CONTROL 0
1141 #define BCM2835_PWM_STATUS 1
1142 #define BCM2835_PWM_DMAC 2
1143 #define BCM2835_PWM0_RANGE 4
1144 #define BCM2835_PWM0_DATA 5
1145 #define BCM2835_PWM_FIF1 6
1146 #define BCM2835_PWM1_RANGE 8
1147 #define BCM2835_PWM1_DATA 9
1150 #define BCM2835_PWMCLK_CNTL 40
1151 #define BCM2835_PWMCLK_DIV 41
1152 #define BCM2835_PWM_PASSWRD (0x5A << 24)
1154 #define BCM2835_PWM1_MS_MODE 0x8000
1155 #define BCM2835_PWM1_USEFIFO 0x2000
1156 #define BCM2835_PWM1_REVPOLAR 0x1000
1157 #define BCM2835_PWM1_OFFSTATE 0x0800
1158 #define BCM2835_PWM1_REPEATFF 0x0400
1159 #define BCM2835_PWM1_SERIAL 0x0200
1160 #define BCM2835_PWM1_ENABLE 0x0100
1162 #define BCM2835_PWM0_MS_MODE 0x0080
1163 #define BCM2835_PWM_CLEAR_FIFO 0x0040
1164 #define BCM2835_PWM0_USEFIFO 0x0020
1165 #define BCM2835_PWM0_REVPOLAR 0x0010
1166 #define BCM2835_PWM0_OFFSTATE 0x0008
1167 #define BCM2835_PWM0_REPEATFF 0x0004
1168 #define BCM2835_PWM0_SERIAL 0x0002
1169 #define BCM2835_PWM0_ENABLE 0x0001
1178 BCM2835_PWM_CLOCK_DIVIDER_2048 = 2048,
1179 BCM2835_PWM_CLOCK_DIVIDER_1024 = 1024,
1180 BCM2835_PWM_CLOCK_DIVIDER_512 = 512,
1181 BCM2835_PWM_CLOCK_DIVIDER_256 = 256,
1182 BCM2835_PWM_CLOCK_DIVIDER_128 = 128,
1183 BCM2835_PWM_CLOCK_DIVIDER_64 = 64,
1184 BCM2835_PWM_CLOCK_DIVIDER_32 = 32,
1185 BCM2835_PWM_CLOCK_DIVIDER_16 = 16,
1186 BCM2835_PWM_CLOCK_DIVIDER_8 = 8,
1187 BCM2835_PWM_CLOCK_DIVIDER_4 = 4,
1188 BCM2835_PWM_CLOCK_DIVIDER_2 = 2,
1189 BCM2835_PWM_CLOCK_DIVIDER_1 = 1
1190 } bcm2835PWMClockDivider;
1193 #ifndef BCM2835_NO_DELAY_COMPATIBILITY
1194 #define delay(x) bcm2835_delay(x)
1195 #define delayMicroseconds(x) bcm2835_delayMicroseconds(x)
bcm2835SPIClockDivider
bcm2835SPIClockDivider Specifies the divider used to generate the SPI clock from the system clock....
@ BCM2835_SPI_CLOCK_DIVIDER_16384
void bcm2835_gpio_clr_aren(uint8_t pin)
volatile uint32_t * bcm2835_clk
void bcm2835_gpio_write(uint8_t pin, uint8_t on)
void bcm2835_spi_transfernb(char *tbuf, char *rbuf, uint32_t len)
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
void bcm2835_aux_spi_transfern(char *buf, uint32_t len)
@ BCM2835_PAD_GROUP_GPIO_0_27
volatile uint32_t * bcm2835_pwm
void bcm2835_gpio_fen(uint8_t pin)
@ BCM2835_SPI_CLOCK_DIVIDER_32768
void bcm2835_pwm_set_range(uint8_t channel, uint32_t range)
void bcm2835_gpio_afen(uint8_t pin)
volatile uint32_t * bcm2835_spi1
uint32_t bcm2835_gpio_eds_multi(uint32_t mask)
void bcm2835_gpio_clr_multi(uint32_t mask)
@ BCM2835_PAD_GROUP_GPIO_28_45
bcm2835SPIChipSelect
bcm2835SPIChipSelect Specify the SPI chip select pin(s)
void bcm2835_aux_spi_writenb(const char *buf, uint32_t len)
void bcm2835_i2c_setClockDivider(uint16_t divider)
uint64_t bcm2835_st_read(void)
void bcm2835_gpio_set_multi(uint32_t mask)
void bcm2835_pwm_set_data(uint8_t channel, uint32_t data)
@ BCM2835_SPI_CLOCK_DIVIDER_65536
volatile uint32_t * bcm2835_st
uint8_t bcm2835_i2c_write_read_rs(char *cmds, uint32_t cmds_len, char *buf, uint32_t buf_len)
@ BCM2835_SPI_CLOCK_DIVIDER_2
uint8_t bcm2835_gpio_eds(uint8_t pin)
void bcm2835_pwm_set_clock(uint32_t divisor)
uint32_t bcm2835_peri_read_nb(volatile uint32_t *paddr)
@ BCM2835_SPI_CLOCK_DIVIDER_1024
void bcm2835_gpio_set_eds(uint8_t pin)
void bcm2835_delayMicroseconds(uint64_t micros)
bcm2835I2CClockDivider
bcm2835I2CClockDivider Specifies the divider used to generate the I2C clock from the system clock....
void bcm2835_spi_end(void)
void bcm2835_gpio_clr(uint8_t pin)
uint8_t bcm2835_spi_transfer(uint8_t value)
uint32_t bcm2835_peri_read(volatile uint32_t *paddr)
RPiGPIOPin
GPIO Pin Numbers.
uint8_t bcm2835_gpio_lev(uint8_t pin)
uint8_t bcm2835_i2c_write(const char *buf, uint32_t len)
@ BCM2835_SPI_BIT_ORDER_MSBFIRST
@ BCM2835_SPI_CLOCK_DIVIDER_32
void bcm2835_pwm_set_mode(uint8_t channel, uint8_t markspace, uint8_t enabled)
void bcm2835_gpio_clr_hen(uint8_t pin)
@ BCM2835_I2C_CLOCK_DIVIDER_148
volatile uint32_t * bcm2835_aux
@ BCM2835_PAD_GROUP_GPIO_46_53
void bcm2835_i2c_end(void)
int bcm2835_aux_spi_begin(void)
uint8_t bcm2835_gpio_get_pud(uint8_t pin)
void bcm2835_spi_setDataMode(uint8_t mode)
uint8_t bcm2835_i2c_read_register_rs(char *regaddr, char *buf, uint32_t len)
void bcm2835_peri_write_nb(volatile uint32_t *paddr, uint32_t value)
void bcm2835_gpio_clr_fen(uint8_t pin)
@ BCM2835_SPI_CLOCK_DIVIDER_64
uint16_t bcm2835_aux_spi_CalcClockDivider(uint32_t speed_hz)
volatile uint32_t * bcm2835_spi0
int bcm2835_i2c_begin(void)
uint8_t bcm2835_i2c_read(char *buf, uint32_t len)
void bcm2835_aux_spi_transfernb(const char *tbuf, char *rbuf, uint32_t len)
uint32_t * bcm2835_regbase(uint8_t regbase)
@ BCM2835_I2C_CLOCK_DIVIDER_150
bcm2835PUDControl
bcm2835PUDControl Pullup/Pulldown defines for bcm2835_gpio_pud()
@ BCM2835_I2C_REASON_ERROR_NACK
@ BCM2835_SPI_CLOCK_DIVIDER_1
int bcm2835_spi_begin(void)
void bcm2835_gpio_ren(uint8_t pin)
uint32_t * bcm2835_peripherals_base
void bcm2835_gpio_clr_ren(uint8_t pin)
void bcm2835_delay(unsigned int millis)
volatile uint32_t * bcm2835_gpio
@ BCM2835_SPI_CLOCK_DIVIDER_4096
@ BCM2835_SPI_CLOCK_DIVIDER_512
@ BCM2835_SPI_CLOCK_DIVIDER_128
void bcm2835_spi_set_speed_hz(uint32_t speed_hz)
void bcm2835_gpio_pudclk(uint8_t pin, uint8_t on)
bcm2835I2CReasonCodes
bcm2835I2CReasonCodes Specifies the reason codes for the bcm2835_i2c_write and bcm2835_i2c_read funct...
@ BCM2835_SPI_BIT_ORDER_LSBFIRST
bcm2835SPIBitOrder
bcm2835SPIBitOrder SPI Bit order Specifies the SPI data bit ordering for bcm2835_spi_setBitOrder()
uint32_t * bcm2835_peripherals
void bcm2835_peri_set_bits(volatile uint32_t *paddr, uint32_t value, uint32_t mask)
@ BCM2835_SPI_CLOCK_DIVIDER_4
bcm2835FunctionSelect
bcm2835PortFunction Port function select modes for bcm2835_gpio_fsel()
void bcm2835_st_delay(uint64_t offset_micros, uint64_t micros)
void bcm2835_spi_write(uint16_t data)
void bcm2835_aux_spi_write(uint16_t data)
void bcm2835_spi_transfern(char *buf, uint32_t len)
void bcm2835_gpio_set(uint8_t pin)
void bcm2835_spi_writenb(const char *buf, uint32_t len)
@ BCM2835_I2C_REASON_ERROR_CLKT
bcm2835SPIMode
SPI Data mode Specify the SPI data mode to be passed to bcm2835_spi_setDataMode()
uint32_t bcm2835_gpio_pad(uint8_t group)
bcm2835RegisterBase
bcm2835RegisterBase Register bases for bcm2835_regbase()
@ BCM2835_SPI_CLOCK_DIVIDER_8192
void bcm2835_gpio_pud(uint8_t pud)
void bcm2835_aux_spi_end(void)
void bcm2835_aux_spi_setClockDivider(uint16_t divider)
volatile uint32_t * bcm2835_pads
void bcm2835_spi_chipSelect(uint8_t cs)
volatile uint32_t * bcm2835_bsc1
void bcm2835_gpio_set_eds_multi(uint32_t mask)
uint32_t bcm2835_peripherals_size
void bcm2835_gpio_len(uint8_t pin)
void bcm2835_gpio_write_mask(uint32_t value, uint32_t mask)
@ BCM2835_I2C_CLOCK_DIVIDER_626
void bcm2835_spi_setChipSelectPolarity(uint8_t cs, uint8_t active)
void bcm2835_i2c_setSlaveAddress(uint8_t addr)
void bcm2835_i2c_set_baudrate(uint32_t baudrate)
void bcm2835_gpio_set_pad(uint8_t group, uint32_t control)
void bcm2835_peri_write(volatile uint32_t *paddr, uint32_t value)
void bcm2835_gpio_aren(uint8_t pin)
@ BCM2835_SPI_CLOCK_DIVIDER_16
@ BCM2835_I2C_CLOCK_DIVIDER_2500
void bcm2835_spi_setBitOrder(uint8_t order)
void bcm2835_gpio_clr_len(uint8_t pin)
void bcm2835_gpio_fsel(uint8_t pin, uint8_t mode)
void bcm2835_spi_setClockDivider(uint16_t divider)
unsigned int bcm2835_version(void)
@ BCM2835_SPI_CLOCK_DIVIDER_256
void bcm2835_set_debug(uint8_t debug)
@ BCM2835_I2C_REASON_ERROR_DATA
@ BCM2835_SPI_CLOCK_DIVIDER_8
void bcm2835_gpio_write_multi(uint32_t mask, uint8_t on)
void bcm2835_gpio_set_pud(uint8_t pin, uint8_t pud)
bcm2835PadGroup
bcm2835PadGroup Pad group specification for bcm2835_gpio_pad()
volatile uint32_t * bcm2835_bsc0
void bcm2835_gpio_clr_afen(uint8_t pin)
@ BCM2835_SPI_CLOCK_DIVIDER_2048
void bcm2835_gpio_hen(uint8_t pin)