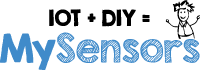 |
MySensors Library & Examples
2.3.2
|
84 const uint8_t EEPROM_ADDR_ERR = 9;
91 TwoWire *communication;
108 extEEPROM(eeprom_size_t deviceCapacity,
byte nDevice,
unsigned int pageSize,
109 byte eepromAddr = 0x50);
113 byte write(
unsigned long addr,
byte *
values,
unsigned int nBytes);
114 byte write(
unsigned long addr,
byte value);
115 byte read(
unsigned long addr,
byte *
values,
unsigned int nBytes);
116 int read(
unsigned long addr);
117 byte update(
unsigned long addr,
byte *
values,
unsigned int nBytes);
118 byte update(
unsigned long addr,
byte value);
123 uint16_t _dvcCapacity;
127 uint16_t _nAddrBytes;
128 unsigned long _totalCapacity;
byte begin(twiClockFreq_t twiFreq=twiClock100kHz, TwoWire *_comm=&Wire)
begin()
unsigned long length()
length()
byte read(unsigned long addr, byte *values, unsigned int nBytes)
read()
byte write(unsigned long addr, byte *values, unsigned int nBytes)
write()
extEEPROM(eeprom_size_t deviceCapacity, byte nDevice, unsigned int pageSize, byte eepromAddr=0x50)
Constructor.
@ twiClock100kHz
twiClock100kHz
byte update(unsigned long addr, byte *values, unsigned int nBytes)
update()
@ twiClock400kHz
twiClock400kHz
Structure to be used in percentage and resistance values matrix to be filtered (have to be in pairs)