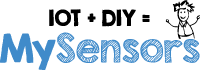 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
49 #include <extEEPROM.h>
53 #ifndef I2CEEPROM_TWI_CLK
54 #define I2CEEPROM_TWI_CLK twiClock400kHz
60 #ifndef I2CEEPROM_PAGE_SIZE
61 #define I2CEEPROM_PAGE_SIZE 64
67 #ifndef I2CEEPROM_CHIP_SIZE
68 #define I2CEEPROM_CHIP_SIZE kbits_256
79 void readBytes(uint32_t addr,
void* buf, uint16_t len);
80 void writeByte(uint32_t addr, uint8_t byt);
81 void writeBytes(uint32_t addr,
const void* buf,
void end()
dummy function for SPI flash compatibility
void blockErase4K(uint32_t address)
dummy function for SPI flash compatibility
uint8_t m_addr
I2C address for busy()
uint8_t readByte(uint32_t addr)
read 1 byte from flash memory
void blockErase32K(uint32_t address)
dummy function for SPI flash compatibility
void writeBytes(uint32_t addr, const void *buf, uint16_t len)
write multiple bytes to flash memory (up to 64K), if define SPIFLASH_SST25TYPE is set AAI Word Progra...
void writeByte(uint32_t addr, uint8_t byt)
Write 1 byte to flash memory.
I2CEeprom(uint8_t addr)
Constructor.
uint16_t readDeviceId()
dummy function for SPI flash compatibility
bool busy()
check if the chip is busy erasing/writing
void wakeup()
dummy function for SPI flash compatibility
void sleep()
dummy function for SPI flash compatibility
void chipErase()
dummy function for SPI flash compatibility
void readBytes(uint32_t addr, void *buf, uint16_t len)
read multiple bytes