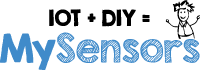 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
74 #include "pins_arduino.h"
79 #ifndef SPIFLASH_WRITEENABLE
80 #define SPIFLASH_WRITEENABLE 0x06
83 #ifndef SPIFLASH_WRITEDISABLE
84 #define SPIFLASH_WRITEDISABLE 0x04
87 #ifndef SPIFLASH_BLOCKERASE_4K
88 #define SPIFLASH_BLOCKERASE_4K 0x20
91 #ifndef SPIFLASH_BLOCKERASE_32K
92 #define SPIFLASH_BLOCKERASE_32K 0x52
95 #ifndef SPIFLASH_BLOCKERASE_64K
96 #define SPIFLASH_BLOCKERASE_64K 0xD8
99 #ifndef SPIFLASH_CHIPERASE
100 #define SPIFLASH_CHIPERASE 0x60
104 #ifndef SPIFLASH_STATUSREAD
105 #define SPIFLASH_STATUSREAD 0x05
108 #ifndef SPIFLASH_STATUSWRITE
109 #define SPIFLASH_STATUSWRITE 0x01
112 #ifndef SPIFLASH_ARRAYREAD
113 #define SPIFLASH_ARRAYREAD 0x0B
116 #ifndef SPIFLASH_ARRAYREADLOWFREQ
117 #define SPIFLASH_ARRAYREADLOWFREQ 0x03
120 #ifndef SPIFLASH_SLEEP
121 #define SPIFLASH_SLEEP 0xB9
124 #ifndef SPIFLASH_WAKE
125 #define SPIFLASH_WAKE 0xAB
128 #ifndef SPIFLASH_BYTEPAGEPROGRAM
129 #define SPIFLASH_BYTEPAGEPROGRAM 0x02
132 #ifndef SPIFLASH_AAIWORDPROGRAM
133 #define SPIFLASH_AAIWORDPROGRAM 0xAD
138 #ifndef SPIFLASH_IDREAD
139 #define SPIFLASH_IDREAD 0x9F
144 #ifndef SPIFLASH_MACREAD
145 #define SPIFLASH_MACREAD 0x4B
156 #ifdef DOXYGEN //needed to tell doxygen not to ignore the define which is actually made somewhere else
157 #define MY_SPIFLASH_SST25TYPE
165 explicit SPIFlash(uint8_t slaveSelectPin, uint16_t jedecID=0);
167 void command(uint8_t cmd,
bool isWrite=
171 void readBytes(uint32_t addr,
void* buf, uint16_t len);
172 void writeByte(uint32_t addr, uint8_t byt);
173 void writeBytes(uint32_t addr,
const void* buf,
193 #ifdef SPI_HAS_TRANSACTION
static uint8_t UNIQUEID[8]
Storage for unique identifier.
bool busy()
check if the chip is busy erasing/writing
void writeByte(uint32_t addr, uint8_t byt)
Write 1 byte to flash memory.
void wakeup()
Wake device.
void readBytes(uint32_t addr, void *buf, uint16_t len)
read unlimited # of bytes
uint16_t readDeviceId()
Get the manufacturer and device ID bytes (as a short word)
void sleep()
Put device to sleep.
void blockErase4K(uint32_t address)
erase a 4Kbyte block
bool initialize()
setup SPI, read device ID etc...
void chipErase()
erase entire flash memory array
uint16_t _jedecID
JEDEC ID.
void blockErase64K(uint32_t addr)
erase a 64Kbyte block
void command(uint8_t cmd, bool isWrite=false)
Send a command to the flash chip, pass TRUE for isWrite when its a write command.
SPIFlash(uint8_t slaveSelectPin, uint16_t jedecID=0)
Constructor.
uint8_t readStatus()
return the STATUS register
uint8_t readByte(uint32_t addr)
read 1 byte from flash memory
void writeBytes(uint32_t addr, const void *buf, uint16_t len)
write multiple bytes to flash memory (up to 64K), if define SPIFLASH_SST25TYPE is set AAI Word Progra...
uint8_t _slaveSelectPin
Slave select pin.
uint8_t * readUniqueId()
Get the 64 bit unique identifier, stores it in UNIQUEID[8].
void blockErase32K(uint32_t address)
erase a 32Kbyte block