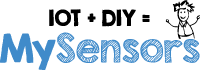 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
20 #ifndef SOFT_I2C_MASTER_H
21 #define SOFT_I2C_MASTER_H
30 #if defined(__AVR__) || defined(DOXYGEN) // AVR only
32 #include <util/delay_basic.h>
76 virtual uint8_t
read(uint8_t
last) = 0;
83 virtual void start() = 0;
89 virtual void stop() = 0;
91 bool transfer(uint8_t addressRW,
void *buf,
92 size_t nbyte, uint8_t option =
I2C_STOP);
94 bool transferContinue(
void *buf,
size_t nbyte, uint8_t option =
I2C_STOP);
117 void begin(uint8_t sclPin, uint8_t sdaPin);
121 bool write(uint8_t b);
126 volatile uint8_t* _sclDDR;
127 volatile uint8_t* _sdaDDR;
128 volatile uint8_t* _sdaInReg;
132 return *_sdaInReg & _sdaBit;
135 void sclDelay(uint8_t n)
140 void writeScl(
bool value)
149 *_sclDDR &= ~_sclBit;
154 void writeSda(
bool value)
163 *_sdaDDR &= ~_sdaBit;
175 template<u
int8_t sclPin, u
int8_t sdaPin>
188 fastDigitalWrite(sclPin,
LOW);
189 fastDigitalWrite(sdaPin,
LOW);
221 if (!fastDigitalRead(sdaPin)) {
261 bool rtn = fastDigitalRead(sdaPin);
270 void sclWrite(
bool value)
272 fastDdrWrite(sclPin, !value);
276 void sdaWrite(
bool value)
278 fastDdrWrite(sdaPin, !value);
282 void readBit(uint8_t bit, uint8_t* data)
286 if (fastDigitalRead(sdaPin)) {
295 void sclDelay(uint8_t n)
301 void writeBit(uint8_t bit, uint8_t data)
303 uint8_t mask = 1 << bit;
304 sdaWrite(data & mask);
312 #endif // SOFT_I2C_MASTER_H
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
const uint8_t STATE_RX_DATA
const uint8_t STATE_TX_DATA
uint8_t read(uint8_t last)
const uint8_t STATE_TX_ADDR_NACK
Two Wire Interface constants.
const uint8_t STATE_TX_DATA_NACK
uint8_t last
8 bit - Id of last node this message passed
AVR Software I2C master class.
const uint8_t STATE_RX_ADDR_NACK
virtual uint8_t read(uint8_t last)=0
AVR Fast software I2C master class.
FW config structure, stored in eeprom.
virtual bool write(uint8_t data)=0
const uint8_t STATE_REP_START
uint8_t read(uint8_t last)
Fast Digital Pin functions.
Base class for FastI2cMaster, SoftI2cMaster.