Contributing Code
The MySensors project is open and welcomes new code contributors. If you plan to make a large refactoring or massive changes, please create an issue first to allow collaborate and provide feedback before submitting a GitHub pull request.. We wouldn't want you to spend a lot of time on something that wouldn't make it into the project.
Fetching the MySensors repository from GitHub
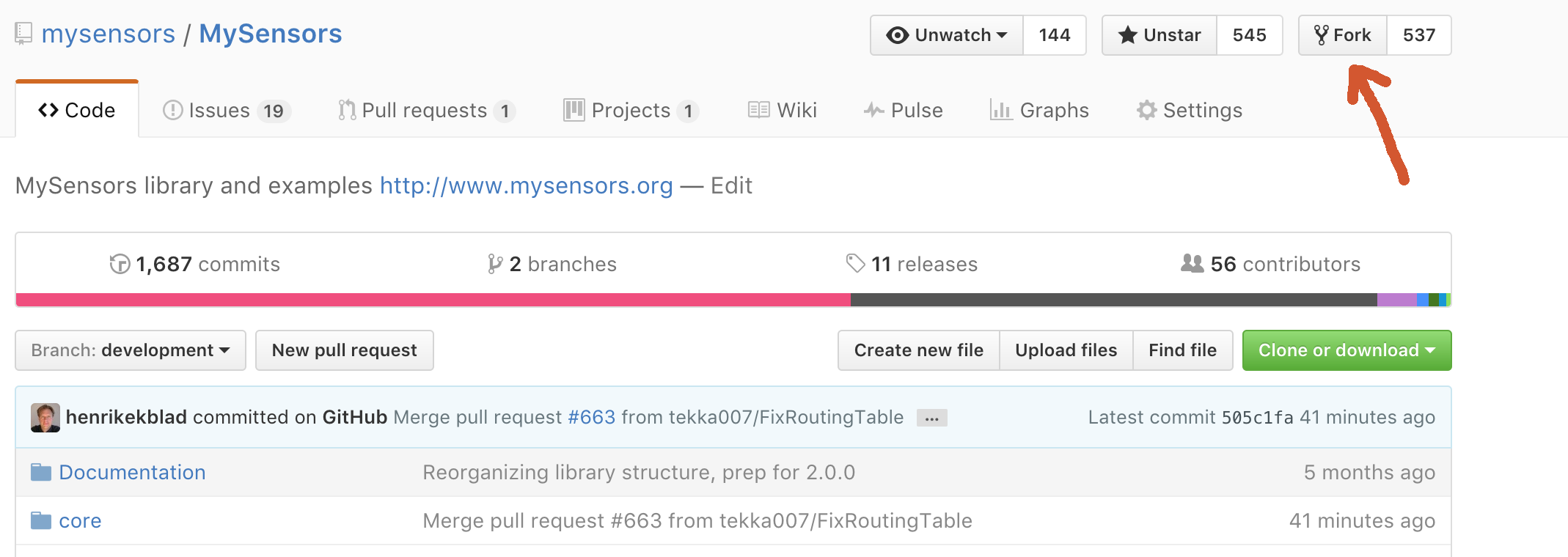
Now it's time to download the forked repository to your computer.
We recommend installing the git command line tool. It's available for all types of platforms.
To fetch the repository from github, run the following command in your terminal window:
# Clone your forked repository to your computer.
> git clone https://github.com/<your username>/MySensors.git
# Change directory to your newly cloned repository
> cd MySensors
# Check out the development branch
MySensors> git checkout development
# Configure your repo for MySensors development (this ensures that you have everything you need to submit a PR)
MySensors> .mystools/bootstrap-dev.sh
NOTE: If bootstrap-dev
prompts you to install required tools, follow the Installation instructions for prerequisite Tools and the re-run .mystools/bootstrap-dev.sh
to complete your repo setup.
Make sure to change your username if prompted above. GitHub also provides Instructions for setting you email address/username
Now you are ready to use your favourite editor or IDE to make the patch and/or enhancement. Fix all warnings when building or the PR won't be accepted otherwise.
When you are ready to submit a PR, make a habit of fetching the latest changes from the upstream repo before committing.
# Fetch upstream changes
MySensors> git fetch upstream
# Merges upstream changes into your local branch
MySensors> git merge upstream/development
# Stage file(s) to for commit to your local repository. Repeat the add command for each changed file or folder you want to commit.
MySensors> git add <file or directory>
# Limit commmit message subject to 50 characters. See "keep a tidy git log" below.
MySensors> git commit -m "Your commmit message"
# Push changes to yor github repository
MySensors> git push
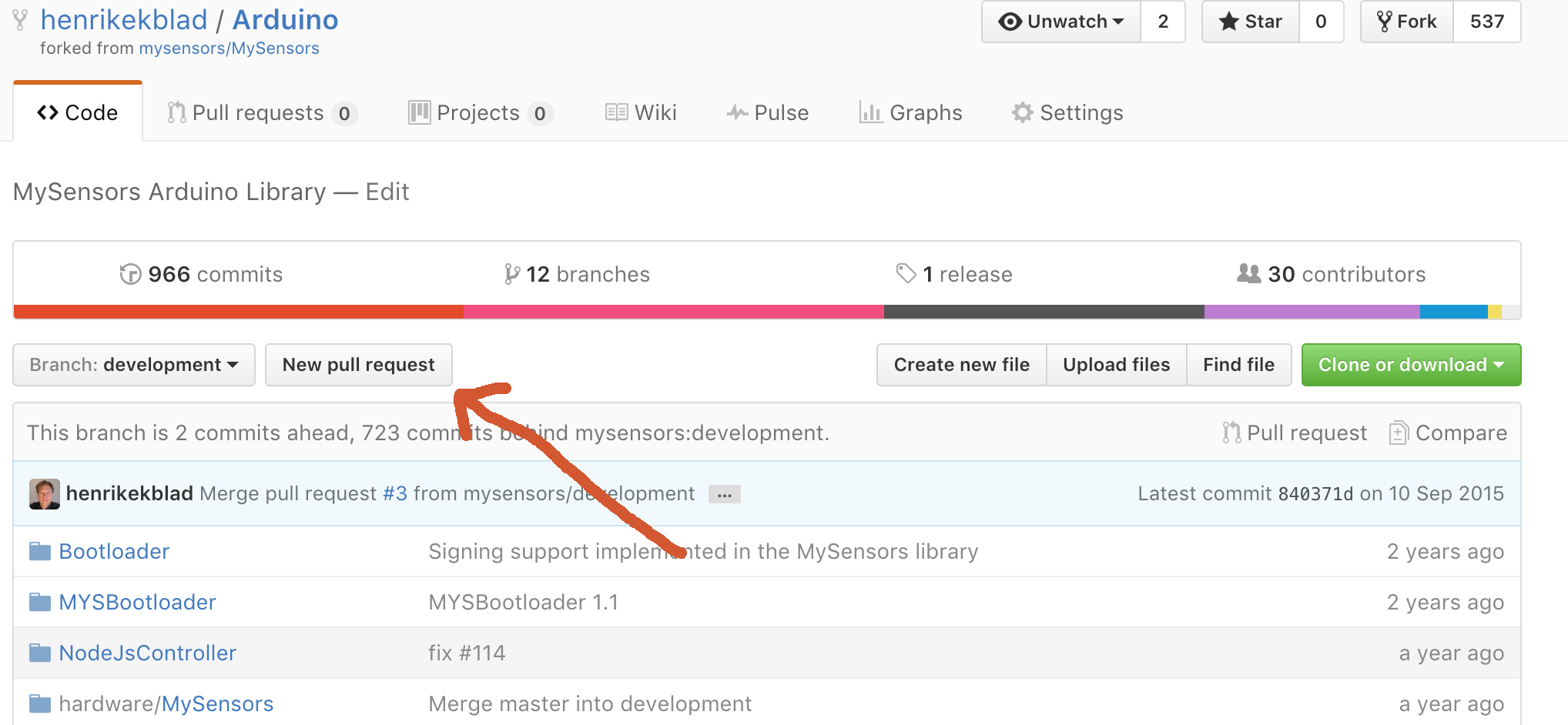
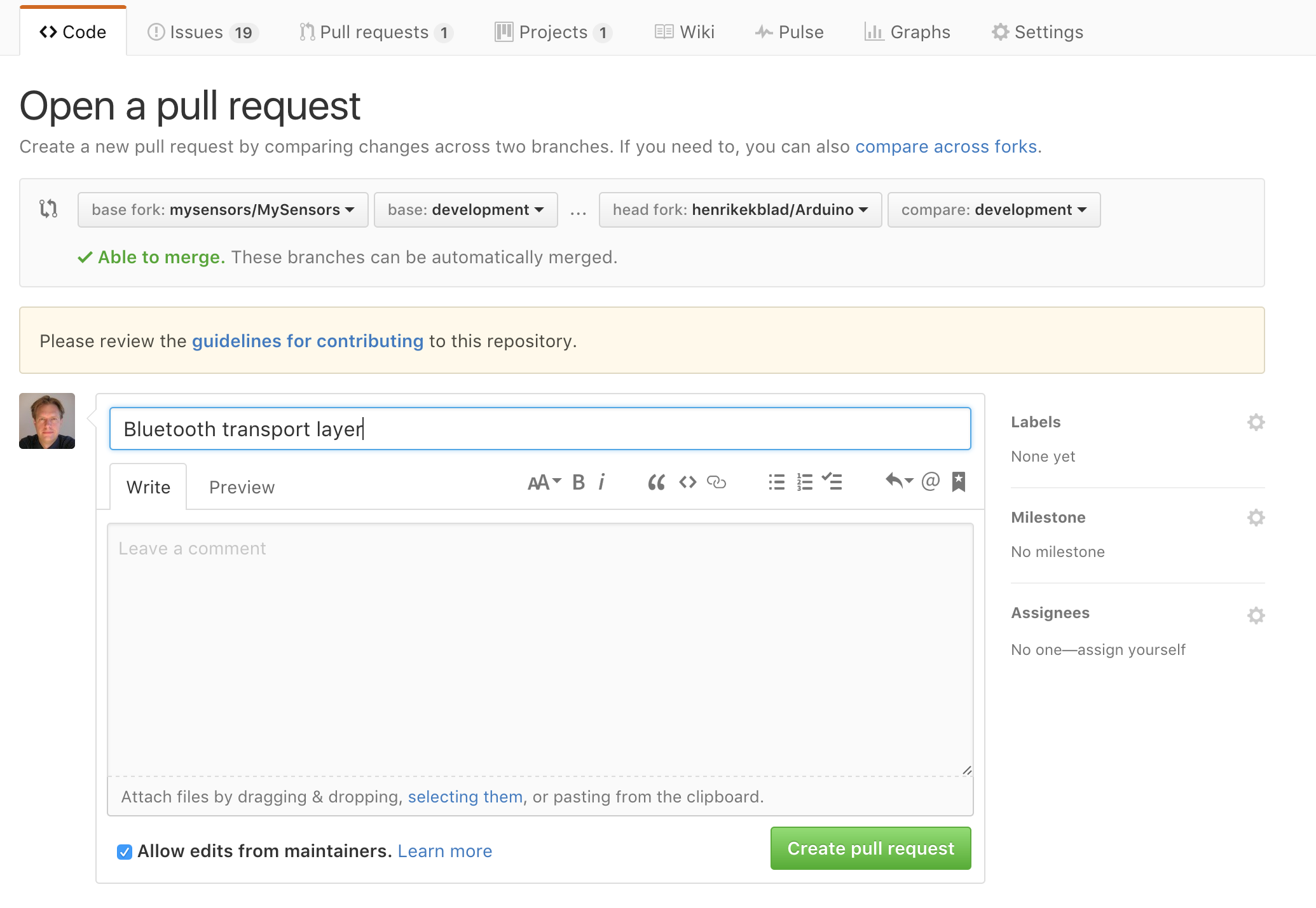
Our build server will run tests on each pull request. It will check the following before accepting it.
- Validity of commit message. Keep subject below 50 chars. Body below 70 chars.
- Codestyle. See Coding Guidelines below.
- No build errors or warnings. We run a series of test builds for different platforms (AVR, ESP8266, SAMD, Linux/RPI). Save yourself a lot of troubles by eliminating warnings before pushing PR.
- Signed Contributor License Agreement (CLA). You can sign it here.
Note! We only accept pull requests on the development branch. Master is only used for releases.
Commit Messages - Keep a tidy git log
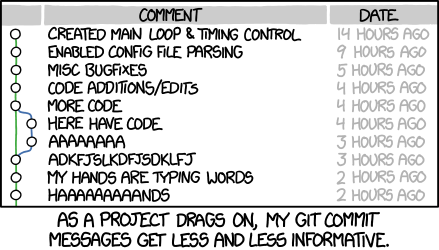
You can find the current MySensors development git log here to get an idea of what a good commit message should look like:
Amending Git Messages or PRs
If your PR gets rejected or if you want to make additions. Amending is the way to go. Followed by a force push. Github will update the PR automatically and our build server will try again.
Rebasing an out-of-date PR
If changes are introduced to the development branch that conflicts with an open PR, the PR might not be possible to merge. You will have to resolve the conflicts and update the PR.
# Fetch upstream changes
MySensors> git fetch upstream
# Merges upstream changes into your local branch
MySensors> git checkout development
MySensors> git merge upstream/development
# Rebase the branch you filed the PR from against your updated development branch
MySensors> git checkout <yourbranch>
MySensors> git rebase development
# You can expect merge conflicts to occur here, which you will need to resolve, add and commit.
# ...
# Force push your updated branch so the PR gets updated
MySensors> git push HEAD:<yourbranch> -f
Signing CLA
To accept contributions to the MySensors core library, you have to sign our contributor license agreement found here: https://www.clahub.com/agreements/mysensors/MySensors
This is not needed, if you just want to add a new example in the examples repository: https://github.com/mysensors/MySensorsArduinoExamples
Coding Guidelines (core library)
Artistic style can be used to verify coding standard and the repository holds a rules definition file at '.astylerc' which can be used to get the rules below verified by the tool. We also offer a git-commit hook to verify the coding structure of the commit in question. It can be installed using 'hooks/install-hooks.sh' and works on Linux, MacOS and Windows (cygwin/bash based shells).
We use camelCase for function and variable names.
Tab-character for white space/indentation.
All control statements ('if', 'for', 'while' etc.) should include curly braces {}. Even one-liners.
Curly braces should follow "One True Brace Style" format like this:
void myFunction() { int i; for (i = 0; i < 42; i++) { if (0 == i) { continue; } } }
All new code should include documentation in doxygen. See an example here.
Avoid using Systems Hungarian notation:
bool bDone; int iCnt;
All lines should be kept at 100 character width or less
- When breaking logical conditionals, keep the conditionals on the first line like this:
if (thisVariable1 == thatVariable1 || thisVariable2 == thatVariable2 || thisVariable3 == thatVariable3)
- When breaking logical conditionals, keep the conditionals on the first line like this:
Preprocessor definitions should be indented if linebroken:
#define Is_Bar(arg,a,b) \ (Is_Foo((arg), (a)) \ || Is_Foo((arg), (b)))
Preprocessor conditions should NOT be indented to source level, but be kept in first column:
#ifdef UNICODE text = wideBuff; #else text = buff; #endif
C++ comments should be indented to source level, and not be kept in first column:
void Foo() { // comment if (isFoo) { bar(); } }
Source files should be stored in UTF-8 encoding with LF/"Unix" style line endings
Trailing whitespaces should be trimmed from all lines
Rules not implemented yet
These rules are not yet enforced (because we need to fix all missing keywords first) but you are very welcome to check if you've added to the backlog.
Keywords in code that don't exist in keywords.txt
SOURCE_FILES="core/ drivers/ hal/ examples/ examples_linux/ MyConfig.h MySensors.h"
for keyword in $(grep -whore 'MY_[A-Z][A-Z_0-9]*' $SOURCE_FILES | sort -u ); do grep -q $keyword keywords.txt || echo $keyword; done
If keywords aren't in keywords.txt, they will not be highlighted in the Arduino IDE. Highlighting makes the code easier to follow and helps spot spelling mistakes.
Keywords in code that don't have Doxygen comments and aren't blacklisted in keywords.txt
time for keyword in $(grep -whore 'MY_[A-Z][A-Z_0-9]*' $SOURCE_FILES | sort -u ); do grep -qE "# $keyword" keywords.txt || git grep -qE "@def $keyword" $SOURCE_FILES || git grep -qE "^#define.*$keyword.*//\!<" $SOURCE_FILES || echo $keyword; done
If keywords don't have Doxygen comments, they will not be available in https://www.mysensors.org/apidocs-beta/index.html Add Doxygen comments to make it easier for users to find and understand how to use the new keywords.