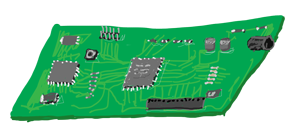
You may run into some weird problems when you start building your own sensors. We've tried to keep a list of common problems and solutions here.
- Are you using the latest stable MySensors library version on EVERY sensor AND the gateway? Does the Controller plugin version match the library version?
- Are you really using a genuine nRF24L01+? Sometimes ebay sellers send out the none-plus version or HopeRF RFM73 clone.
- Triple-check the radio wiring.
- Check the power to the radio module. It requires a stable 3.3V power source. Try adding a decoupling capacitor over the 3.3V and GND pins to minimize the ripple. Choose a cap around 47uF with low ESR if possible. Check polarity of capacitor. Or switch to a ceramic variant.
- Try switching to another power source. Some cheap USB chargers give very poor output.
- Powering your sensor through your computers USB port? Make sure it has enough juice. Especially when using amplified radio version.
- Keep leads between Arduino and radio short. If possible, skip the dupont cables and mount things closely on a prototype pcb board.
- If you're using an amplified version of the NRF24L01+ chip (with an external antenna) and experience communication problems you could have problem feeding it with enough power. Try bypassing Arduinos regulator by feeding it directly from a 3.3v power source. You can also try adjusting (lowering) the PA level. This is done by adding parameters to the begin() method or editing MyConfig.h before uploading sketch to the problematic sensor node. Note that PA level does not affect the receive sensitivity. See the MySensors Library API for more information. This radio can also have problems with shielding and an external shield may need to be added.
- Some have reported big differences in reception based on the orientation of the radio.
Enabling Debug and Monitoring the Logs
To enable debug messages in the examples and the gateway, you need just add #define MY_DEBUG in the sketch before including MySensors.h.
After you have enabled debugging, connect your sensor or gateway via USB to your computer (like you are uploading a sketch). From the Arduino IDE, select the correct Port from the Tools menu then open the Serial Monitor to view the debug messages. Note: the default Baud Rate for MySensors is 115200
The sensor will print debug messages when sending and receiving data over the radio network. This if very useful information for diagnosing a problem in your setup.
In Summary:
- Build and upload the sketch with the above debug flag enabled in MyConfig.h.
- Keep the sensor connected to your computer and enable the Serial Monitor in the Arduino IDE. Tools>Serial Monitor.
- Set the baud-rate to 115200.
Debug message format
Debug messages will look like this:
0;255;3;0;9;MCO:BGN:INIT GW,CP=RNNGA--,VER=2.0.1-beta
0;255;3;0;9;TSM:INIT
0;255;3;0;9;TSM:INIT:TSP OK
0;255;3;0;9;TSM:INIT:GW MODE
0;255;3;0;9;TSM:READY
IP: 192.168.10.80
0;255;3;0;9;MCO:REG:NOT NEEDED
0;255;3;0;9;MCO:BGN:STP
0;255;3;0;9;MCO:BGN:INIT OK,ID=0,PAR=0,DIS=0,REG=1
0;255;3;0;9;TSF:MSG:READ,12-4-0,s=18,c=1,t=16,pt=1,l=1,sg=0:1
0;255;3;0;9;TSF:MSG:READ,12-4-0,s=31,c=1,t=16,pt=1,l=1,sg=0:1
You can use our online Log Parser to interpret the content.
The serial protocol used between the Gateway and the Controller is a simple semicolon separated list of values. The last part of each "command" is the payload. All commands ends with a newline. The serial commands has the following format (see the Serial Protocol documentation for more info):
node-id ; child-sensor-id ; message-type ; ack ; sub-type ; payload \n
0;0;3;0;9 means node 0 , sensor 0, internal message (3), not an ack message (0), log message (9). This means that it's the gateway that prints this info as a log after already having received the message from a node.
The Transport messages (TSM & TSF) are described here.
The MySensors Core messages (MCO) are described here
Here is how to interpret the alphanumeric codes at the end of the sent/received messages. For example: s=31,c=1,t=16,pt=1,l=1,sg=0:1
s = sensor id
c = message type 0-4: presentation, set, req, internal or stream. See link
t = value type: S_VALUE during presentation or V_VALUE during set/req
pt = payload type: string, byte int, etc. See link
l = message length
sg = signed or unsigned message: 0 or 1 for false or true
Clearing EEPROM
Your sensors contains a small static memory (EEPROM). This will hold information about the unique id of your sensor and routing information. On rare occasions you might need to clear this memory back to the factory default.
To clear the EEPROM memory, upload the following sketch to your sensor or gateway (and wait 20 seconds).
/*
* The MySensors Arduino library handles the wireless radio link and protocol
* between your home built sensors/actuators and HA controller of choice.
* The sensors forms a self healing radio network with optional repeaters. Each
* repeater and gateway builds a routing tables in EEPROM which keeps track of the
* network topology allowing messages to be routed to nodes.
*
* Created by Henrik Ekblad <[email protected]>
* Copyright (C) 2013-2019 Sensnology AB
* Full contributor list: https://github.com/mysensors/MySensors/graphs/contributors
*
* Documentation: http://www.mysensors.org
* Support Forum: http://forum.mysensors.org
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* version 2 as published by the Free Software Foundation.
*
*******************************
*
* DESCRIPTION
*
* This sketch clears radioId, relayId and other routing information in EEPROM back to factory default
*
*/
// load core modules only
#define MY_CORE_ONLY
#include <MySensors.h>
void setup()
{
Serial.begin(MY_BAUD_RATE);
Serial.println("Started clearing. Please wait...");
for (uint16_t i=0; i<EEPROM_LOCAL_CONFIG_ADDRESS; i++) {
hwWriteConfig(i,0xFF);
}
Serial.println("Clearing done.");
}
void loop()
{
// Nothing to do here...
}
More Troubleshooting Tips
You can get more troubleshooting tips here or by watching the following video.